For this project I was in charge of the enemy AI. I'll be sharing the interesting stuff I did and how the enemy works.
Waypoints
The first thing I had to do was make an enemy that had to go on patrol. The enemy had to move towards a waypoint, and if it reached said waypoint, he had to move on to the next one. He had to also turn towards the waypoint, because otherwise it would only face one direction. This is what it looks like in script.

Following the player
I struggled with this the most. Just following waypoints is one thing, but going after the player when it gets close is a whole other thing. There where a number of things I had to to work on.
1.Make the enemy follow the player closest to him.
2.Have the enemy keep a distance from the player.
3.Make the enemy align with the player's x or y position.
4.Not have the enemy object go askew.
5.Not have the enemy sprite face the wrong direction.
6.Make the enemy go after the next player if the first one dies.
There were mere points that I got stuck on, like fixing the sprite animation, but those are arguably less interesting to talk about.
First point 1. I had to find out how to get the closest player. This is how it looks like in script.
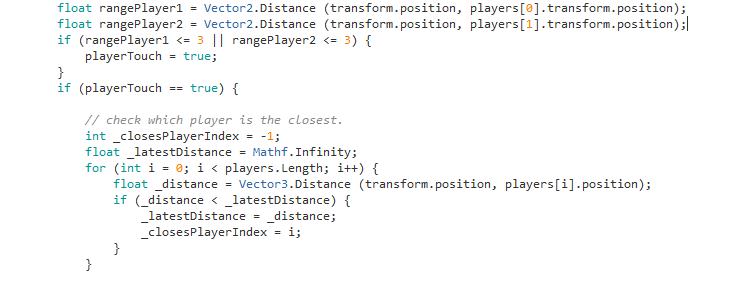
I first had to make two variables calculating the distance between the enemy and player one and the enemy and two named rangePlayer1 and rangePlayer2 respectively. When one of these players gets close it activates the script that follows the player instead of the waypoints. The first thing the follow script does is find out which player is the closest. It calculates the closest player index so I can use it for the rest of the script.
Now for point 2. When I first started I didn't even know if there was a way to keep distance the way I wanted it. Luckily I had some help and it worked out greatly.

The first thing I had to do was normalise (change the magnitude to 1) the distance between the enemy and the player, so I can change it later. After that I defined the distance. After that I defined the target the enemy has to move towards, calculated by adding the normalised distance, multiplying it with the distance minus a set number I can change so I can create a greater distance, and adding it to the target position.
Point 3. Make the enemy align with the player's x or y position. For this I attached four gameobjects to each player, defining the top, bottom, left and right of both players.

After that I had to look where my enemy was positioned in accordance to the player and use define my quadrants and use that to change my target accordingly.

Once I know which quadrant I'm on I use that to define the current point (cp) and use that to change the target and improve the script.

As you can see I replaced the closest player index with cp.
if (transform.position.x != players[_closesPlayerIndex].transform.position.x && transform.position.y != players[_closesPlayerIndex].transform.position.y)
this activates the script if the X and Y positions don't align.
Point 4, don't make the enemy object go askew. For this I had to round up my current angle divided by 90 . And use the rounded up number and multiply it by 90. this gives me a round up number of my angles.
Example : (147.3/90) = 1.636. Round it up to 2.
2 * 90 = 180. 180 is the angle that the player is turned now.

Point 5, make the sprite face the right way. Because of the way I handled my player movement my sprites were turned in weird ways. to fix this I flipped the sprites around to make them face the right way. Not the best way to fix things, but I had to do what I had to do.


Point 6. Make the enemy go after the next player if the first one dies. For this I checked which player died and changed the closest player index accordingly.

These were the interesting tidbits of my AI I wanted to share. Please have a look at the work of my fellow teammates.